Bitmap graphics
Moscrif supports two different types of image processing - bitmap and vector. This article describes how to define bitmap object and how to display it at the mobile device screen in Moscrif. Bitmap is kept as image file and represents complex picture, which is hard to create in vector format. With using bitmap, it is much easier to create the appearance of “natural” media, such as areas of watercolours bleeding into each other. Bitmaps can be read and modified by any bitmap-based software and stored in certain file formats.
Moscrif supports the most popular ones :
- .jpeg - format uses lossy compression but does not support alpha channel. It is frequently used for digital photography.
- .png - bitmap format of image that employs loss less data compression. This format also support alpha channel
Bitmaps cannot be rescaled up or down to an arbitrary resolution without loss of apparent quality.
Bitmap definition
To define a Bitmap object from an image file in Moscrif, we use fromFile. Once bitmap object is created, basic properties such as width and height are available.
To get image size without loading an image file we use getSize. Method returns pair of values. The first value is width and second is height.
Bitmap drawing
Moscrif native Canvas class supports four different ways of how to draw Bitmap object at the mobile device screen.
- drawBitmap
- drawBitmapMatrix
- drawBitmapRect
- drawBitmapNine
Canvas.drawBitmap - common function for drawing a picture from an image file anchored at the defined position in origin resolution of the image.
include "lib://uix/application.ms"
var app = new Application();
app.onStart = function(sender)
{
//load bitmap into memory
this.bitmap = Bitmap.fromFile("app://logo.png");
}
app.onDraw = function(sender, canvas)
{
//draw bitmap to the center of the window
var x = System.width / 2 - this.bitmap.width / 2;
var y = System.height / 2 - this.bitmap.height / 2;
canvas.drawBitmap(this.bitmap, x, y);
}
app.run();
Canvas.drawBitmapMatrix function - draws bitmap object with transformation (rotation, skew, scale etc.) defined by instance of Matrix class.
include "lib://uix/application.ms"
var app = new Application();
app.onStart = function(sender)
{
//load bitmap into memory
this.bitmap = Bitmap.fromFile("app://logo.png");
this.matrix = new Matrix();
//move bitmap to the middle of screen
var cx = System.width / 2 - this.bitmap.width / 2;
var cy = System.height / 2 - this.bitmap.height / 2;
this.matrix.setTranslate(cx, cy);
//rotate bitmap to 90° cw around the image center
this.matrix.preRotate(90, cx, cy);
}
app.onDraw = function(sender, canvas)
{
//draw bitmap to the center of the window
canvas.drawBitmapMatrix(this.bitmap, this.matrix);
}
app.run();
Canvas.drawBitmapRect function that allows to place more images into one target object at the screen, mostly used in the game development. It loads only a part of the image, defined as source rectangle and displays it into defined target rectangle.
include "lib://uix/application.ms"
var app = new Application();
app.onStart = function(sender)
{
// loads bitmap into memory
this.bitmap = Bitmap.fromFile("app://logo.png");
// define source rectangle
// loads only left half of the image
this.sourceRect = {
left: 0,
top: 0,
right: this.bitmap.width / 2,
bottom: this.bitmap.height
}
// define target rectangle in the center of screen
// and loads only left half of the image
this.targetRect = {
left: System.width / 2 - this.bitmap.width / 4,
top: System.height / 2 - this.bitmap.height / 2,
right: System.width / 2 + this.bitmap.width / 4,
bottom: System.height / 2 + this.bitmap.height / 2
}
}
app.onDraw = function(sender, canvas)
{
// draw only left half of source image
canvas.drawBitmapRect(this.bitmap,
this.sourceRect.left, this.sourceRect.top,
this.sourceRect.right, this.sourceRect.bottom,
this.targetRect.left, this.targetRect.top,
this.targetRect.right, this.targetRect.bottom);
}
app.run();
Canvas.drawBitmapNine function that draws whole image to target rectangle. This function expands only the centre of the image, which is useful for drawing some objects; e.g. button.
Object rendered by Canvas.drawBitmapNine does not need to adjust an appearance of the graphics in the corners. Function defines source bitmap object and position on the screen.
Example of the buttons – one normal size and the other one from the same source, but stretched to expected width:
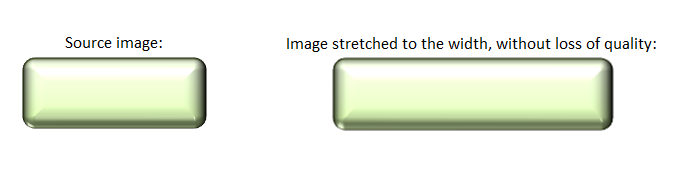
include "lib://uix/application.ms"
var app = new Application();
app.onStart = function(sender)
{
// loads bitmap into memory
this.bitmap = Bitmap.fromFile("app://logo.png");
// calculate image position on the screen
this.targetRect = {
left: 0,
top: System.height / 2 - this.bitmap.height / 2,
right: System.width,
bottom: System.height / 2 + this.bitmap.height / 2,
}
}
app.onDraw = function(sender, canvas)
{
//draw bitmap stretched to the width
canvas.drawBitmapNine(this.bitmap,
this.targetRect.left, this.targetRect.top,
this.targetRect.right, this.targetRect.bottom);
}
app.run();
Using Canvas.drawBitmapNine function with an instance of the Paint class (what defines Paint.maskFilter and Paint.colorFilter), we can create some effects like convert to grey scale, blur effect etc.