Canvas
Canvas class encapsulates all states of drawing into a smart mobile device. This includes a reference to the device itself and a stack of matrix/clip values. For any given draw call (e.g. drawRect), the geometry of the object being drawn is transformed by the concatenation of all the matrices in the stack. The transformed geometry is clipped by the intersection of all of the clips in the stack. While the Canvas holds the state of the drawing device, the state (style) of the object being drawn is held by Paint, which is provided as a parameter in each of the draw methods. Paint holds attributes such as colour, typeface, textSize, strokeWidth, shader (e.g. gradients, patterns), etc.
Moscrif is designed to render graphics objects up to 60 fps. If system idle allows to do onDraw, Moscrif refreshes Canvas (screen). Moscrif rendering functionality guarantees 40 fps for each smart mobile device.
In other words, Moscrif executes onDraw method every 25 milliseconds, if system idle allows it. Therefore it is highly recommended to prepare all graphics and text objects outside of onDraw method, due to performance issue.
Let's have a look to some examples of canvas use in Moscrif.
Canvas.Translate - this method changes current matrix centre point to co-ordinates within method parameters.
app.onDraw = function(sender, canvas)
{
canvas.clear(0xff000000);
// move center of canvas from left top corner to center of the center of screen
canvas.translate(System.width / 2, System.height / 2);
// draw bitmap to the center of screen
canvas.drawBitmap(this._img, this._img.width / -2, this._img.height / -2);
}
Canvas.contact - this method concatenates current matrix with Matrix set as parameter.
app.onDraw = function(sender, canvas)
{
// create matrix
var m = new Matrix();
// apply more transformations (translate and rotation)
m.setTranslate(System.width / 2, System.height / 2);
// rotate 45° clockwise
m.preRotate(45);
// apply matrix to canvas
canvas.concat(m); // draw bitmap
canvas.drawBitmap(this._img, this._img.width / -2, this._img.height / -2);
}
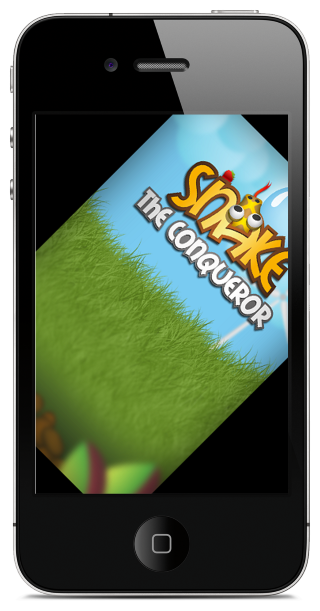
Canvas.clipRect - this method modifies the current clip with the specified rectangle. Parameters specify the position of image on the screen and region options.
Region options are :
- #difference - Subtracts the op region from the first region.
- #intersect - Intersects the two regions.
- #union - Union (inclusive-or) the two regions.
- #xor - Exclusive-or the two regions.
- #reverseDifference -Subtracts the first region from the op region.
- #replace - Replaces the dst region with the op region.
app.onDraw = function(sender, canvas)
{
//clipRect with region option intersect
canvas.clipRect(System.width / 4, System.height / 4, 3 * System.width / 4, 3 * System.height / 4, #intersect );
canvas.drawBitmap(this._img, 0, 0);
//clipRect with region option difference
canvas.clipRect(System.width / 4, System.height / 4, 3 * System.width / 4, 3 * System.height / 4, #difference );
canvas.drawBitmap(this._img, 0, 0);
}
Canvas.drawPoints, this method draws series of points, based on pointMode. Location of the points is defined as array and JSON object with x co-ordinate and y co-ordinate. Drawing can be changed by instance of Paint.
Point mode options can be :
- #points - DrawPoints draws each point separately.
- #lines - DrawPoints draws each pair of points as a line segment.
- #polygon - DrawPoints draws the array of points as a polygon.
app.onDraw = function(sender, canvas)
{ canvas.clear(0xffffffff);
var paint = new Paint();
paint.strokeWidth = 15;
paint.strokeCap = #round; var pts = new Array({x: 100, y: 100}, {x: 150, y: 150}, {x: 50, y: 150});
canvas.drawPoints(#points, pts, paint); pts = new Array({x: 100, y: 200}, {x: 150, y: 250}, {x: 50, y: 250}, {x: 100, y: 200});
canvas.drawPoints(#polygon, pts, paint); pts = new Array({x: 100, y: 300}, {x: 150, y: 350}, {x: 150, y: 350}, {x: 50, y: 350}, {x: 50, y: 350}, {x: 100, y: 300});
canvas.drawPoints(#lines, pts, paint); }
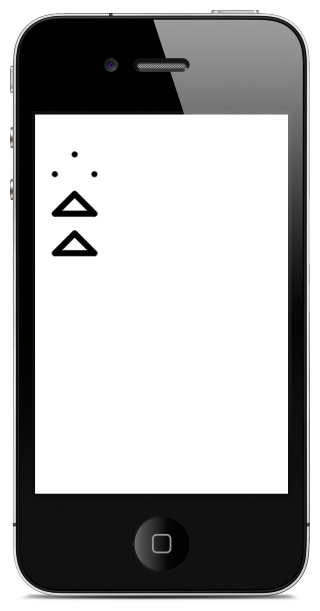
See full definition of Canvas class in Moscrif API Docs.