Text is drawn by drawText function, which has for parameters and the last parameter is an instance of Paint class. The paint object allows applying onto text same effects as on all other graphics objects.
Gradients
Gradients are created by Shader class. Moscrif supports three types of gradient: linear, sweep and bitmap. The last mentioned, bitmap gradient is used to fill the text (also all other graphics shapes) by texture. The detail sample of how to use gradient is Gradients sample.
Note: Functions from shader class, take some coordinates as their parameters. In game framework, these coordinates are calculated for the whole screen not only the drawn shape. By default, the coordinates [0, 0] are in the left top corner of the screen. In application framework, coordinates are calculated for every element like button, form, list item etc. the coordinates [0, 0] in the left top corner of object.
Example: create linear gradient
// LINEAR GRADIENT
this._paintLG = new Paint();
var pts = {
start: {x: 0, y: System.height / 8},
end: {x: 0, y: System.height / 4},
};
//define array of colors (red, green, blue) in hexadecimal format [canal + RGB].
var clrs = new Array(0xffff0000, 0xff00ff00, 0xff0000ff);
// define array of position
var pos = new Array(0, 0.5, 1);
// creates shader of linear gradient
var shader = Shader.linear(pts, clrs, pos, 0);
this._paintLG.shader = shader;
this._paintLG.textSize = System.height / 8;
Example: create sweep gradient
// SWEEP GRADIENT
this._paintSG = new Paint();
//define array of colors: red, green, blue (canals: alpha, red, green, blue in hexadecimal form)
var colors = new Array(0xffff0000, 0xff00ff00, 0xff0000ff);
//define array of position
pos = new Array(0, 0.5, 1);
//creates shader of sweet gradient, first two parameters are positioned in the center on X and y axis, then color palette, and position for every color
this._paintSG.shader = Shader.sweep(System.width / 2, System.height / 2, colors, pos);
this._paintSG.textSize = System.height / 8;
Example: create bitmap gradient
// BITMAP GRADIENT
this._paintBG = new Paint();
//define array of colors: red, green, blue (canals: alpha, red, green, blue in hexadecimal form)
this._paintBG.shader = Shader.bitmap( "app://texture.png", #clamp, #clamp);
this._paintBG.textSize = System.height / 8;
When shaders are applied onto paint objects, the texts can be easily drawn using these paints.
Example: draw texts
game.onDraw = function(sender, canvas)
{
canvas.clear(0xffffffff);
// draw text with linear gradient
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, System.height / 4, this._paintLG);
// draw text with sweep gradient
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, (System.height + h) / 2, this._paintSG);
// draw text with bitmap gradient (texture)
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, 3*System.height / 4, this._paintBG);
}
Image: linear, sweep and bitmap gradient
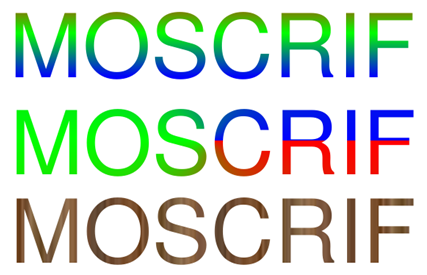
Other effects
In addition to gradients that Moscrif supports other graphics effects like mask or color filter are supported also. Mask filter allows creating blur by blur method. Final blur effect made by blur method can be customized by the method's parameters, which specifies the radius and type of blur effect.
NOTE: Blur method has large requirements on device performance. It should be used very carefully and it also should not be animated.
Example: create paints with various blur effects
var texture = Shader.bitmap("app://texture.png", #clamp, #clamp);
this._paintA = new Paint();
this._paintA.textSize = System.height / 8;
this._paintA.shader = texture;
// create MaskFilter and apply it to the paint object
this._paintA.maskFilter = MaskFilter.blur(20, #inner); this._paintB = new Paint();
this._paintB.textSize = System.height / 8;
this._paintB.shader = texture;
// create MaskFilter and apply it to the paint object
this._paintB.maskFilter = MaskFilter.blur(20, #outer); this._paintC = new Paint();
this._paintC.textSize = System.height / 8;
this._paintC.shader = texture;
// create MaskFilter and apply it to the paint object
this._paintC.maskFilter = MaskFilter.blur(20, #solid); this._paintD = new Paint();
this._paintD.textSize = System.height / 8;
this._paintD.shader = texture;
// create MaskFilter and apply it to the paint object
this._paintD.maskFilter = MaskFilter.blur(20, #normal);
When mask filters are applied onto paint objects, the texts can be easily drawn using these paints.
game.onDraw = function(sender, canvas)
{
canvas.clear(0xffffffff); canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, System.height / 5, this._paintA);
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, 2*System.height / 5, this._paintB);
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, 3*System.height / 5, this._paintC);
canvas.drawText("MOSCRIF", (System.width - this._textWidth)/2, 4*System.height / 5, this._paintD);
}
Image: various blug effects
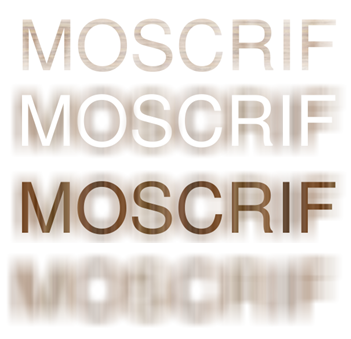