Moscrif SDK offers box2D physics engine. Box2d allows 2D physical simulation. Working with box2D is really easy, because it is designed to emulate real world. This article explains only native (no framework) classes and functions.
Box2D uses units from SI system for physical quantities (meters, kilograms, and seconds) and radians for angle.
To achieve smaller size of final program; by default box2d native libraries are not included in the project. It is necessary to add it via Project preferences->application dialog.
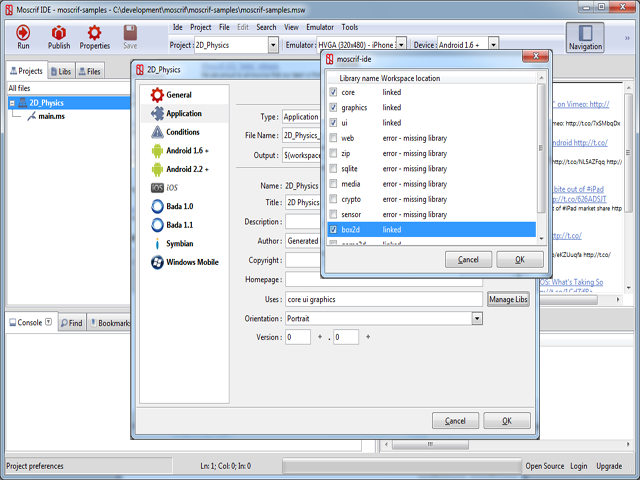
Physics world
is managed by b2World class. This is a basic class which draws and manages all other physical objects. This class contains b2World.doDraw and b2World.doDebugDraw; both used to draw physical world. This function is usually called within app.onDraw event.
Another important function is b2World.step, that is used to take a time step. Time step determines how to move physical objects between two calls of this function. Collision detection, integration, and constraint solution are also performed during this step. This function is usually called within onProcess function and time step is set to 1.0/40.0, which is the shortest time between two calls of onProcess function.
When two world’s bodies collide, b2World.onBeginContact function is called. The function has two parameters that specify collision. The first is sender (object which called this function) and second is an instance of b2Contact. b2Contact object contains information such as bodies which collided, or physical properties of collision. When the collision is finished, b2World.onEndContact is called.
Joints, bodies and real world properties like gravity (b2World. setGravity) are managed by this class as well.
var app = new Moscrif.Window()
app._terminate = false; app.onStart = function(sender)
{
// create new physical world
this.world = new b2World();
} app.onProcess = function(sender)
{
// take a time step to perform collision detection, integration, and constraint solution.
this.world.step(1.0/40.0);
// redraw screen
app.invalidate();
return this._terminate ? 0 : 1;
}
app.onDraw = function(sender, canvas)
{
// fill background with black color
canvas.clear(0xff000000);
// draw physical world
this.world.doDraw(canvas);
} app.init(true).run();
Bodies
Bodies are created by b2World.createBody function according to required shape. Function returns instance of b2Body. Shape of body is determined by b2CircleShape or b2PolygonShape class. Second parameter is body type:
#static |
A static body behaves as if it has infinite mass. It does not move under simulation and can only be moved manually. A static body has zero velocity and does not collide with other static or kinematic bodies. |
#kinematic |
A kinematic body moves under simulation according to its velocity. Kinematic bodies do not respond to forces. They can be moved manually by the user, but normally a kinematic body is moved by setting its velocity. A kinematic body behaves as if it has infinite mass, but it does not collide with other static or kinematic bodies. |
#dynamic |
A dynamic body is fully simulated. They can be moved manually by PhysicsBody.setPosition(), but normally they move according to forces. A dynamic body can collide with all body types and always has finite, non-zero mass. If you try to set the mass of dynamic body to zero, it will automatically acquire a mass of one kilogram. |
Other physical properties (e.g. density, friction or bounce) and default x and y position can be set in the function as well. Another way of how to move a body is by using b2Body. setPosition and b2Body.setTransform. Naturally, bodies (according to theirs type) move under physical simulation. Using setPosition and setTransform may cause non-physical behavior.
B2Body class offers many physical activities (force applying, linear impulse applying etc.). When redraw is needed, body b2Body.onDraw() function is called.
// create new physical world
this.world = new b2World(); // create body shape
var shape = new b2CircleShape(10/*radius*/);
// create new circle body
var body = this.world.createBody(shape, #dynamic, 1.0, 1.0, 1.0, System.width / 2, System.height / 2);
// set drawing function
body.onDraw = function(sender, canvas)
{
//get position of circle
var (left, top) = sender.getPosition(); //draw green circle
var paint = new Paint();
paint.color = 0xff00ff00; // chanels: alpha, red, green, blue
canvas.drawCircle(left.toInteger(), top.toInteger(), 10, paint);
}
Joints
Joints are used to constrain bodies to the world or to each other. Typical examples in games include ragdolls, teeters, and pulleys. Joints can be combined in many different ways to create interesting motions.
Some joints provide limits so you can control the range of motion. Some joint provide motors which can be used to drive the joint at prescribed speed until prescribed force/torque is reached. Moscrif offers eight types of joints: distance (b2DistanceJoint), friction (b2FrictionJoint), gear (b2GearJoint), mouse (b2MouseJoint), prismatic (b2PrismaticJoint), pulley (b2PulleyJoint), revolute (b2RevoluteJoint) and weld (b2WeldJoint). Every pne of these joints have different behavior and properties. Joints are created by b2World.createDistanceJoint, b2World.createFrictionJoint, b2World.createGearJoint etc.