Moscrif implements four basic scene transitions with sliding effect into top, bottom, left or right.
• SlideToTop, slide current scent to Top
• SlideToBottom, slide current scent to Bottom
• SlideToLeft, slide current scent to Left
• SlideToRight, slide current scent to Right
All scene transition classes above are extended from Game2D.
Scene Transition:
It specifies generic public attributes like duration, transition, sceneFrom and sceneTo. Event SceneTransitions.onComplete is fired when transition is finished.
Animation effect is done via Moscrif Animator object defined in SceneTransitions._start() method.
/** Start transition
@private
*/
function _start() {
var duration = this._duration;
var transition = this._transition;
var onComplete = this._onCompleteHanlder;
var animator = new Animator({duration: duration, transition: transition, onComplete: onComplete});
animator.addSubject(this)
animator.play();
}
Example below shows usage of all scene transitions. There is a scene with background picture and four GameButtons Top, Bottom, Left and Right. Each button's onCLick event does scene sliding effect to desired direction.
include "lib://game2d/game.ms"
include "lib://game2d/scene.ms"
include "lib://game2d/textButton.ms"
include "lib://game2d/sceneTransitions/slideToLeft.ms";
include "lib://game2d/sceneTransitions/slideToRight.ms";
include "lib://game2d/sceneTransitions/slideToTop.ms";
include "lib://game2d/sceneTransitions/slideToBottom.ms"; var game = new Game(); //define sample scene and scene buttons
game.onStart = function(sender)
{
this._background = Bitmap.fromFile("app://menu_back.png"); //game scene instance
var scene = new Scene();
scene.draw = function(canvas)
{
canvas.drawBitmap(this super._background, 0, 0);
super.draw(canvas);
} this.push(scene); //text decoration object
var textBold = new Paint();
textBold.textSize = System.height/20;
textBold.typeface = Typeface.fromName("mailrays", #bold);
textBold.color = 0xffffffff; //create buttons
var btnTop = new TextButton({text: "Top", paint: textBold,x:System.width/2,y:System.height/2+System.height/15});
btnTop.onClick = function() {
//scene transition to top
this super.push(scene, new SlideToTop({duration:1000,transition:Animator.Transition.bouncy}));
};
scene.add(btnTop); var btnBottom = new TextButton({text: "Bottom", paint: textBold,x:System.width/2,y:System.height/2+System.height/15*2});
btnBottom.onClick = function() {
//scene transition to Bottom
this super.push(scene, new SlideToBottom({duration:1000,transition:Animator.Transition.bouncy}));
};
scene.add(btnBottom); var btnLeft = new TextButton({text: "Left", paint: textBold,x:System.width/2,y:System.height/2+System.height/15*3});
btnLeft.onClick = function() {
//scene transition to Left
this super.push(scene, new SlideToLeft({duration:1000,transition:Animator.Transition.bouncy}));
};
scene.add(btnLeft); var btnRight = new TextButton({text: "Right", paint: textBold,x:System.width/2,y:System.height/2+System.height/15*4});
btnRight.onClick = function() {
//scene transition to Right
this super.push(scene, new SlideToRight({duration:1000,transition:Animator.Transition.bouncy}));
};
scene.add(btnRight); } //run sample
game.run();
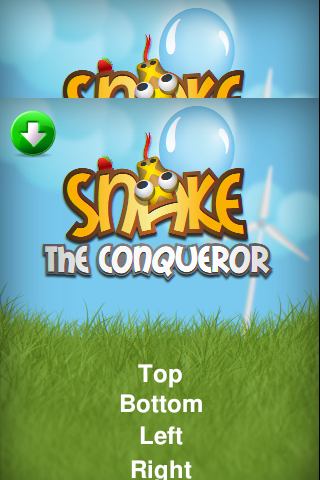
Picture: Move Current scene to the Bottom