This animation smoothly darkens background and increases photo size.
Image 1: show photo
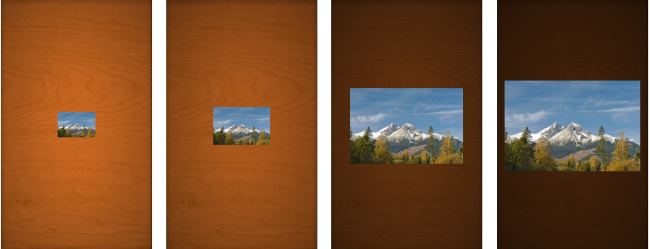
Image 2: hide photo
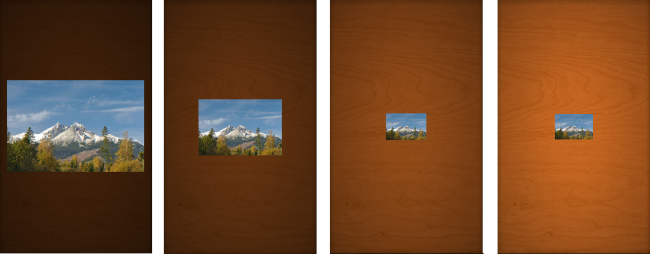
To create this effect I used Moscrif game framework and animator class. Every photo is represented by one instance of PhotoSprite, which is derived from Sprite class. Sprite class impalements image drawing. Only what we need to do is draw rectangle under the photo, which darkens background.
Example code 1: draw function
function draw(canvas)
{
// draw black rectangle up to background
canvas.drawRect(0, 0, System.width, System.height, this._bgPaint); // draw image
super.draw(canvas);
}
Only what we need to do in animation function is change alpha level of background and also scale property of image. This causes nice animation.
Example code 2: animator - display photo
function show()
{
this._showed = true;
// keep scale of preview // show photo
var showPhoto = new Animator ({
duration : 800,
transition : Animator.Transition.strongEasyOut,
});
showPhoto.addSubject(function(state)
{
this super.scale = this super._previewScale + (1.0 - this super.previewScale)*state;
});
showPhoto.play(); // darken background
var fadeBg = new Animator ({
duration : 800,
transition : Animator.Transition.strongEasyOut,
});
fadeBg.addSubject(function(state)
{
this super.bgPaint.alpha = (170*state).toInteger();
});
fadeBg.play();
}
Example code 3: animator - hide photo
function hide()
{
this._showed = false;
// keep scale of preview // hide photo
var showPhoto = new Animator ({
duration : 800,
transition : Animator.Transition.strongEasyOut,
});
showPhoto.addSubject(function(state)
{
this super.scale = this super._previewScale + (this super.scale - this super.previewScale)*state;
});
showPhoto.reverse(); // lighten background
var fadeBg = new Animator ({
duration : 800,
transition : Animator.Transition.strongEasyOut,
});
fadeBg.addSubject(function(state)
{
this super.bgPaint.alpha = (170*state).toInteger();
});
fadeBg.reverse();
}
Complete code is available in here. When you combine this blog with previous you would be able to create same photo gallery as is well known from web.