While application responds, it is good habit to show some loading animation on the screen. In the next sample I will show you how to create this animation in Moscrif. Of course, it is only one type of animation and you can adapt it to your requirements.
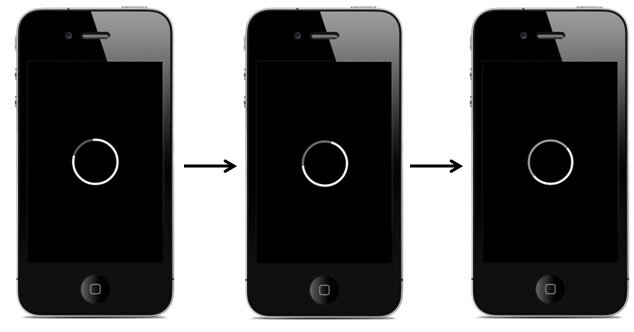
Main parts of this element are two animators:
- First animator which changes color of background:
this._colorAnimator = new Animator({ duration : 750,
transition: Animator.Transition.strongEasyIn,
});
this._colorAnimator.addSubject(function(state)
{
this super._bgCirclePaint.alpha = (state * 230).toInteger();
});
this._colorAnimator.onComplete = function()
{
this super._bgCirclePaint.alpha = 0;
this.play();
}
this._colorAnimator.play();
- And second which changed size of slider:
this._animator = new Animator({
duration : 750,
transition: Animator.Transition.linear,
});
this._animator.addSubject(function(state)
{
this super._angle = ((state)*360).toInteger();
this super._redrawSlider();
});
this._animator.onComplete = function()
{
this super._finalPosition -= 165;
this super._angle = 360;
this super._redrawSlider();
this.reverse();
} this._animator.reverse();
Conclusion
Project file
include "lib://game2d/game.ms" include "app://loader.ms" var game = new Game(); game.onStart = function()
{
var loader = new Loader({height : 100, width : 100, x : System.width / 2, y : System.height / 2}); var scene = new Scene();
scene.add(loader); game.push(scene);
} game.run();
Loader class
include "lib://game2d/base/gameControl.ms" include "lib://core/animation/animator.ms" class Loader : GameControl
{
function init()
{
super.init(); this._path = new Path();
this._angle = 360;
this._finalPosition = -25; this._createAnimators();
this._createPaints();
} property height(v)
{
get return this._height;
set {
this._height = v;
}
} property width(v)
{
get return this._width;
set {
this._width = v;
}
} property x(v)
{
get return this._x;
set {
this._x = v;
}
} property y(v)
{
get return this._y;
set {
this._y = v;
}
} function _createPaints()
{
this._sliderPaint = new Paint();
this._sliderPaint.style = #stroke;
this._sliderPaint.strokeWidth = 5;
this._sliderPaint.color = 0xffffffff;
this._bgCirclePaint = new Paint();
this._bgCirclePaint.style = #stroke;
this._bgCirclePaint.strokeWidth = 5;
this._bgCirclePaint.color = 0xffffffff;
this._bgCirclePaint.alpha = 0;
this._bgCircle = new Paint();
this._bgCircle.style = #stroke;
this._bgCircle.strokeWidth = 5;
this._bgCircle.color = 0xff4c4c4c;
} function _createAnimators()
{
this._animator = new Animator({
duration : 750,
transition: Animator.Transition.linear,
});
this._animator.addSubject(function(state)
{
this super._angle = ((state)*360).toInteger();
this super._redrawSlider();
});
this._animator.onComplete = function()
{
this super._finalPosition -= 165;
this super._angle = 360;
this super._redrawSlider();
this.reverse();
}
this._animator.reverse(); this._colorAnimator = new Animator({
duration : 750,
transition: Animator.Transition.strongEasyIn,
});
this._colorAnimator.addSubject(function(state)
{
this super._bgCirclePaint.alpha = (state * 230).toInteger();
});
this._colorAnimator.onComplete = function()
{
this super._bgCirclePaint.alpha = 0;
this.play();
}
this._colorAnimator.play();
} function _redrawSlider()
{
this._path.reset();
this._path.arcTo(this.x - this.width / 2, this.y - this.height / 2, this.x + this.width / 2, this.y + this.height / 2, this._finalPosition - this._angle / 2, this._angle, true);
} function draw(canvas)
{
canvas.clear(0xff000000);
canvas.drawOval(this.x - this.width / 2, this.y - this.height / 2, this.x + this.width / 2, this.y + this.height / 2, this._bgCircle);
canvas.drawOval(this.x - this.width / 2, this.y - this.height / 2, this.x + this.width / 2, this.y + this.height / 2, this._bgCirclePaint);
canvas.drawPath(this._path, this._sliderPaint);
}
}
Download project here!