Probably, the most interesting is how to create suspensions. To create it I used prismatic joint with limits and motors. The motor has maximum force, which allows depressing suspensions if it hits some barrier.
The car is created in create function of Car class. The car consists from car chassis, front and back damper and wheels.
Example: create all bodies
function create(scene, x, y)
{
var damperHeight = 8*this._images.wheel.width * this._scale/10;
var wheelDistance = 7 * this._images.body.width*this._scale / 10; // create bodies:
this._body = scene.addPolygonBody(this._images.body, #dynamic, 0.1, 0.0, 0.0, this._images.body.width*this._scale, this._images.body.height*this._scale);
this._body.z = 2;
this._body.scale = this._scale;
this._body.setPosition(x, y);
// FRONT
this._frontDamper = scene.addPolygonBody(null, #dynamic, 10.0, 0.0, 0.0, 2, this._images.wheel.width / 2 * this._scale);
this._frontDamper.setPosition(x + wheelDistance / 2, y + damperHeight - this._images.wheel.width / 4 * this._scale); this._frontWheel = scene.addCircleBody(this._images.wheel, #dynamic, 0.1, 0.4, 0.0, this._images.wheel.width / 2 * this._scale);
this._frontWheel.scale = this._scale;
this._frontWheel.setPosition(x + wheelDistance / 2, y + damperHeight); // BACK
this._backDamper = scene.addPolygonBody(null, #dynamic, 10.0, 0.0, 0.0, 2, this._images.wheel.width / 2 * this._scale);
this._backDamper.setPosition(x - wheelDistance / 2, y + damperHeight - this._images.wheel.width / 4 * this._scale); this._backWheel = scene.addCircleBody(this._images.wheel, #dynamic, 0.1, 0.4, 0.0, this._images.wheel.width / 2 * this._scale);
this._backWheel.scale = this._scale;
this._backWheel.setPosition(x - wheelDistance / 2, y + damperHeight);
The bodies are connected together by joints. The prismatic joint connects suspensions and revolute joint connects wheels. The revolute joint also supports joint motor, what allows to rotate the wheels.
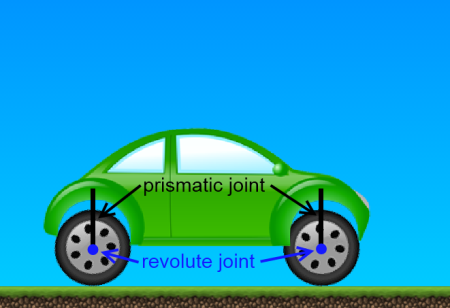
Example: create joints
// JOINTS
// prismatic joins
var jointDef = {
lowerTranslation : -3 * (damperHeight / scene.scale) / 10, //(damperHeight / 5) / scene.scale, /*meters*/
upperTranslation : 0.0, /*meters*/
enableLimit : true,
enableMotor : true,
motorSpeed : 2.5,
maxMotorForce : this._body.getMass() * 8.5,
}
this._joints.push(scene.createPrismaticJoint(this._frontDamper, this._body, x + wheelDistance / 2, y, 0.0, 1.0, 0.0, jointDef, false));
this._joints.push(scene.createPrismaticJoint(this._backDamper, this._body, x - wheelDistance / 2, y, 0.0, 1.0, 0.0, jointDef, false)); // revolute joints
jointDef = {
enableMotor : true, // enable motor
maxMotorTorque : 1500000, // maximum torque
motorSpeed : 0.0, // it is changed latery*/
}
// frontDamper.fixedRotation = true;
// backDamper.fixedRotation = true;
this._motorJoint = scene.createRevoluteJoint(this._frontDamper, this._frontWheel, x + wheelDistance / 2, y + damperHeight, jointDef, false);
this._motorJointB = scene.createRevoluteJoint(this._backDamper, this._backWheel, x - wheelDistance / 2, y + damperHeight, jointDef, false); this._joints.push(this._motorJoint);
this._joints.push(this._motorJointB);
this._joints.push(scene.createMouseJoint(this._body, this._frontWheel, null, false));
this._joints.push(scene.createMouseJoint(this._body, this._backWheel, null, false));
}
You can see the result on following video.
Detailed tutorial how to do it will be soon published on Moscrif’s web page or codeproject side.
Source code is free available here!
CodeProject