Few days ago, I have created Breakout game for Android, iOS and Bada (using Moscrif). Of course, this old game is not interesting enough for today’s users. In addition to dividing bricks to four different levels I have also added some amazing animations. Our version of game is placed into futuristic space. To make paddle more interesting, I added an effect of electrical spark onto it.
This effect consists from four images: paddle without effect and then three pictures with effect. Two of these images are used twice. Five images are more than enough, because electricity effect does not require high fps rate. We use five frames in 500ms animation.
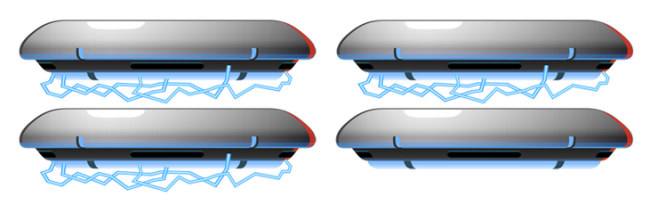
To achieve realistic appearance of animation, it is connected with sound.
Firstly, all source images are loaded using:
game._loadResources = function()
{
this._eletricShockImages = new Array(Bitmap.fromFile("app://disk.png"), Bitmap.fromFile("app://disk1.png"), Bitmap.fromFile("app://disk3.png"), Bitmap.fromFile("app://disk2.png"), Bitmap.fromFile("app://disk1.png"), Bitmap.fromFile("app://disk3.png"));
this._wavElectric = new AudioPlayer();
this._wavElectric.openFile("app://electricshock.wav");
}
Timing of animation is managed by Timer object. When the game starts, timer is set to random value between 1,5 to 3 seconds. To make the effect more realistic, animation is played in random time intervals.
game._eletricShock = function(img = 0)
{
if (img == 1)
this._wavElectric.play();
this._paddle.image = this._eletricShockImages[img];
img++;
if (img == 6)
img = 0; this._timer = new Timer(100, false); // interval make no sense here
if (img != 1)
this._timer.start(100);
else
this._timer.start(1500 + rand(1500));
this._timer.onTick = function(sender) {this super._eletricShock(img)};
}
Following code shows this effect. Complete code of Breakout game is available in Breakout demo.
include "lib://game2d/game.ms" include "lib://box2d/physicsWorld.ms" var game = new Game(); game.onStart = function()
{
this._loadResources(); // create physics world
// Physics world with small gravitation (0.5 m/s^2)
this._world = new PhysicsWorld(0.0, -0.5, true, true); // paddle position
this._paddleY = System.height - (System.height / 5);
this._paddleX = System.width / 2;
// load image
this._imgPaddle = Bitmap.fromFile("app://disk.png");
this._paddle = this._world.addPolygonBody(this._imgPaddle, #static, 0.0, 0.0, 1.05, this._imgPaddle.width, this._imgPaddle.height);
this._paddle.setPosition(this._paddleX, this._paddleY); // set timer, which starts animation
this._timer = new Timer(10000, false); // interval make no sense here
this._timer.start(1500 + rand(1000));
this._timer.onTick = function(sender) {this super._eletricShock(1)};
} game.onDraw = function(sender, canvas)
{
canvas.clear(0xff000000);
// draw physics world
this._world.doDraw(canvas);
} game._loadResources = function()
{
this._eletricShockImages = new Array(Bitmap.fromFile("app://disk.png"), Bitmap.fromFile("app://disk1.png"), Bitmap.fromFile("app://disk3.png"), Bitmap.fromFile("app://disk2.png"), Bitmap.fromFile("app://disk1.png"), Bitmap.fromFile("app://disk3.png"));
this._wavElectric = new AudioPlayer();
this._wavElectric.openFile("app://electricshock.wav");
} game._eletricShock = function(img = 0)
{
if (img == 1)
this._wavElectric.play();
this._paddle.image = this._eletricShockImages[img];
img++;
if (img == 6)
img = 0; this._timer = new Timer(100, false); // interval make no sense here
if (img != 1)
this._timer.start(100);
else
this._timer.start(1000 + rand(1500));
this._timer.onTick = function(sender) {this super._eletricShock(img)};
} game.run();
Download project